top of page
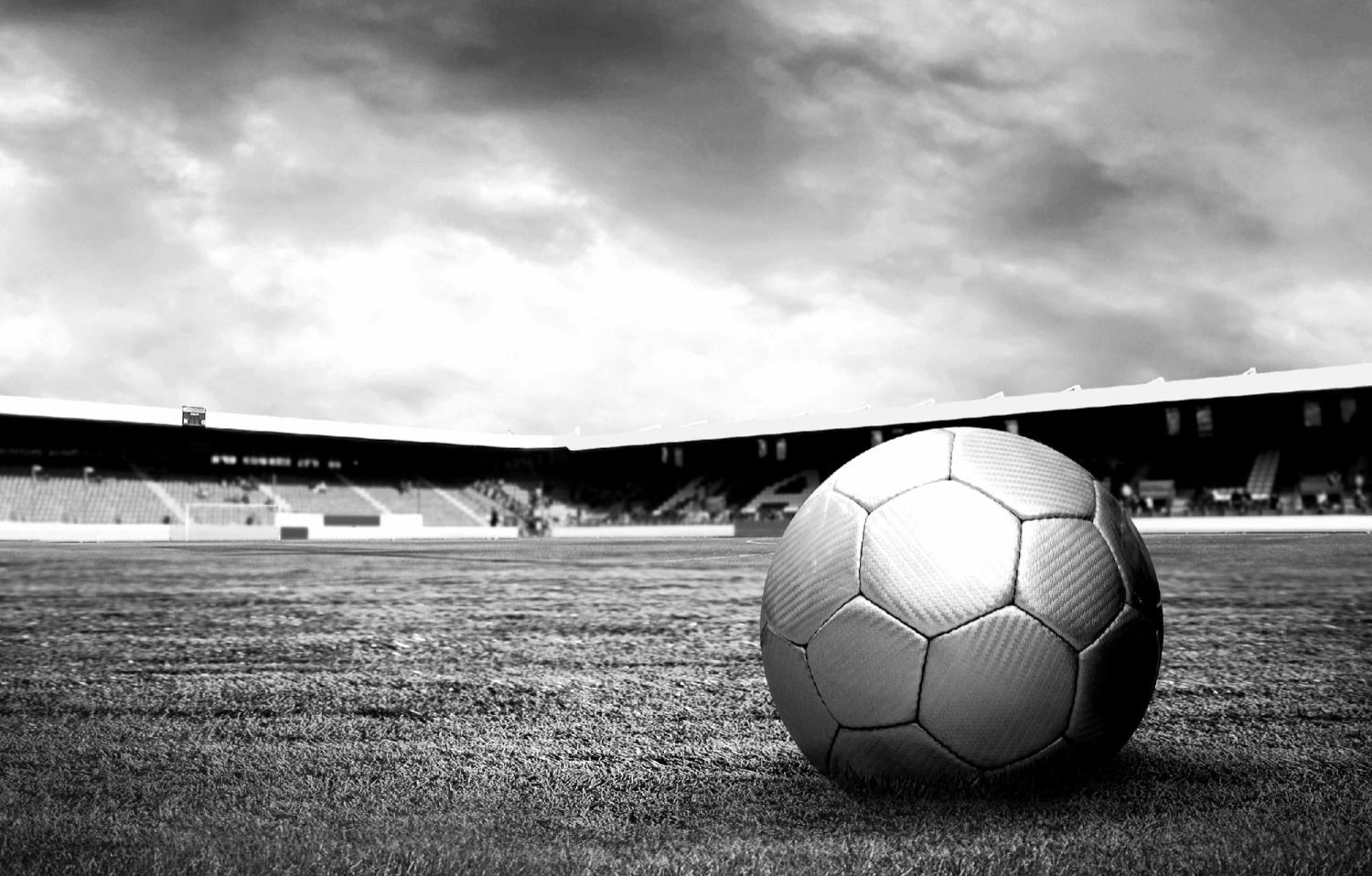
Dedicated to the Scientific Study of the Antique Automobile, Its Accessories, Archives, and Romantic Lore

Team Discussions
Public·86 members
Post Not Found
It seems like this post was deleted
bottom of page
Dedicated to the Scientific Study of the Antique Automobile, Its Accessories, Archives, and Romantic Lore
Post Not Found
It seems like this post was deleted